Stack class in java is a Last In First Out (LIFO) data structure.Β Stack is a subclass of Vector which implements a last-in first-out stack.
Stack class in java supports two important operations named asΒ pushΒ andΒ pop.
TheΒ pop
Β operation removes an item from the top of the stack, while, theΒ push
Β operation adds an item at the top of the stack.
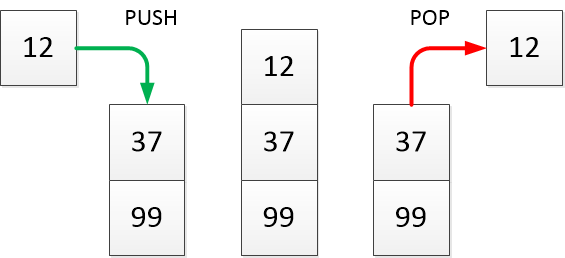
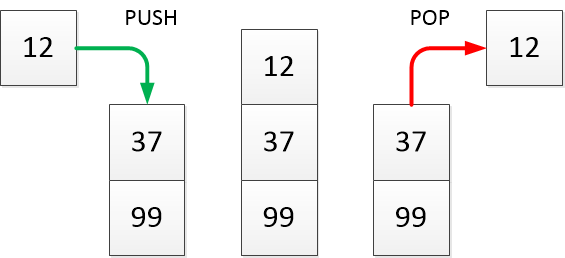
How Stack is implemented in Java
Stack class in java is linear data structure which follows LIFO method.
Java Collection framework has a Stack class which represents and implements Stack data structure.
Stack class in java is subclass of Vector class.
Following is the hierarchy of Stack class in Java –
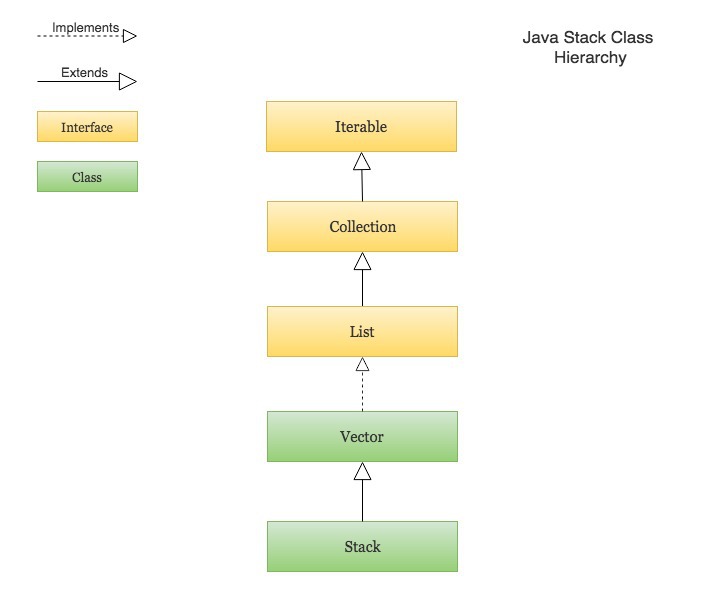
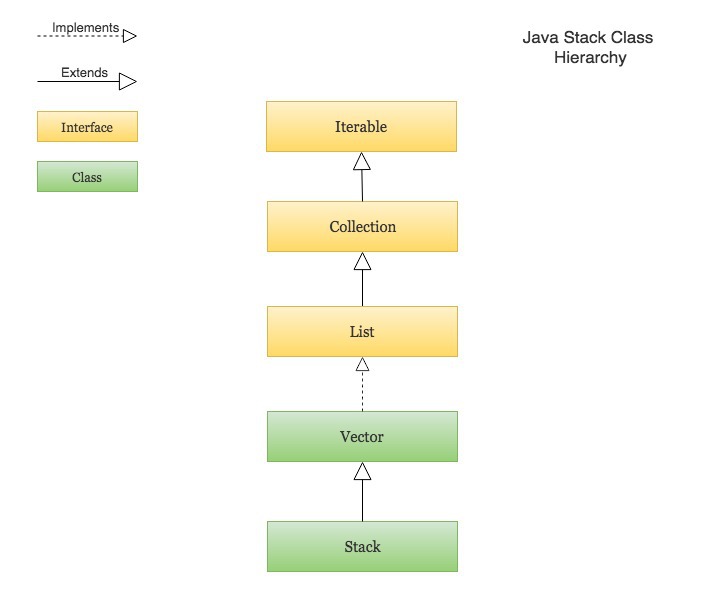
Stack class extendsΒ Vector
class, it grows and shrinks its size as when new elements are added or removed respectively.
Stack class has following methods apart from the methods inherited from its parent class Vector.
# | Method & Description |
---|---|
1 | boolean empty() Checks if the stack is empty. And returns true if the stack is empty and returns false if the stack has elements. |
2 | Object peek( ) Returns the item on the top of the stack, but it does not remove it. |
3 | Object pop( ) Returns the item on the top of the stack, but it removes the element. |
4 | Object push(Object element) Pushes the element on the top of stack and element is returned. |
5 | int search(Object element) Searches for element in the stack. If it is found, offset from the top of the stack is returned. Otherwise, -1 is returned. |
Stack Push and Pop operation
Below is the example of Stack Push and Pop operations
import java.util.Stack; public class StackDemoExample { public static void main(String[] args) { // Creating a Stack Stack<String> stackOfDisc = new Stack<>(); // Pushing new items to the Stack stackOfDisc.push("D1"); stackOfDisc.push("D2"); stackOfDisc.push("D3"); stackOfDisc.push("D4"); System.out.println("Stack => " + stackOfDisc); System.out.println(); // Popping items from the Stack String discAtTop = stackOfDisc.pop(); // Throws EmptyStackException if the stack is empty System.out.println("Stack.pop() => " + discAtTop); System.out.println("Current Stack => " + stackOfDisc); System.out.println(); // Get the item at the top of the stack without removing it discAtTop = stackOfDisc.peek(); System.out.println("Stack.peek() => " + discAtTop); System.out.println("Current Stack => " + stackOfDisc); } }
Output:-- Stack => [D1, D2, D3, D4] Stack.pop() => D4 Current Stack => [D1, D2, D3] Stack.peek() => D3 Current Stack => [D1, D2, D3]
Important Stack Operations
- Finding the size of the stack
- Search for an element in the Stack
- Check if the stack is empty
import java.util.Stack; public class StackDemoExample { public static void main(String[] args) {Stack<String> stackOfDiscs = new Stack<>(); stackOfDiscs.push("D1"); stackOfDiscs.push("D2"); stackOfDiscs.push("D3"); stackOfDiscs.push("D4"); System.out.println("Stack : " + stackOfDiscs); // Check if the Stack is empty System.out.println("Is Stack empty? : " + stackOfDiscs.isEmpty()); // Finding the size of Stack System.out.println("Size of Stack : " + stackOfDiscs.size()); // Search for an element // The search() method returns the 1-based position of the element from the top of the stack // It returns -1 if the element was not found in the stack int position = stackOfDiscs.search("D2"); if(position != -1) { System.out.println("Found the element \"D2\" at position : " + position); } else { System.out.println("Element not found"); } } }
Output:-- Stack : [D1, D2, D3, D4] Is Stack empty? : false Size of Stack : 4 Found the element "D2" at position : 3
Iterating over a Stack
- Iterate over a Stack using iterator().
- Iterate over a Stack using iterator() and Java 8 forEachRemaining() method.
- Iterate over a Stack from Top to Bottom using listIterator().
- Iterate over a Stack using Java 8 forEach().
import java.util.Iterator; import java.util.ListIterator; import java.util.Stack; public class StackDemoExample { public static void main(String[] args) { Stack<String> stackOfDiscs = new Stack<>(); stackOfDiscs.add("D1"); stackOfDiscs.add("D2"); stackOfDiscs.add("D3"); stackOfDiscs.add("D4"); System.out.println("=== Iterate over a Stack using Java 8 forEach() method ==="); stackOfDiscs.forEach(plate -> { System.out.println(plate); }); System.out.println("\n=== Iterate over a Stack using iterator() ==="); Iterator<String> platesIterator = stackOfDiscs.iterator(); while (platesIterator.hasNext()) { String plate = platesIterator.next(); System.out.println(plate); } System.out.println("\n=== Iterate over a Stack using iterator() and Java 8 forEachRemaining() method ==="); platesIterator = stackOfDiscs.iterator(); while (platesIterator.hasNext()) { String plate = platesIterator.next(); System.out.println(plate); } System.out.println("\n=== Iterate over a Stack from TOP to BOTTOM using listIterator() ==="); // ListIterator allows you to traverse in both directions. ListIterator<String> platesListIterator = stackOfDiscs.listIterator(stackOfDiscs.size()); while (platesListIterator.hasPrevious()) { String plate = platesListIterator.previous(); System.out.println(plate); } } }
Output:-- === Iterate over a Stack using Java 8 forEach() method === D1 D2 D3 D4 === Iterate over a Stack using iterator() === D1 D2 D3 D4 === Iterate over a Stack using iterator() and Java 8 forEachRemaining() method === D1 D2 D3 D4 === Iterate over a Stack from TOP to BOTTOM using listIterator() === D4 D3 D2 D1
Conclusion
In this article, you have learned what is a Stack and how to create a Stack in Java, perform push and pop operations in a Stack, check whether the Stack is empty or not, finding the size of the Stack and ways to iterate over Stack of elements. We have explained with java stack examples.