Overview
In this tutorial, we are going to explain the various ways of How to write to a file in Java with the illustrative examples.
Java write to file line by line is often needed in our day to day projects for creating files through java. There are various classes present in Java which can be used for write to file line by line.
Letβs look at them :
Java write to file line by line using FileOutputStream class
FileOutputStream class is an output stream used for writing data to a File or FileDescriptor. FileOutputStream class is used to write streams of raw bytes such as image data. This class is good to use with bytes of data which cannot be represented as text such as excel document, PDF files, image files etc.
FileOutputStream class is part of the java.io package and it is a subclass of OutputStream
class.
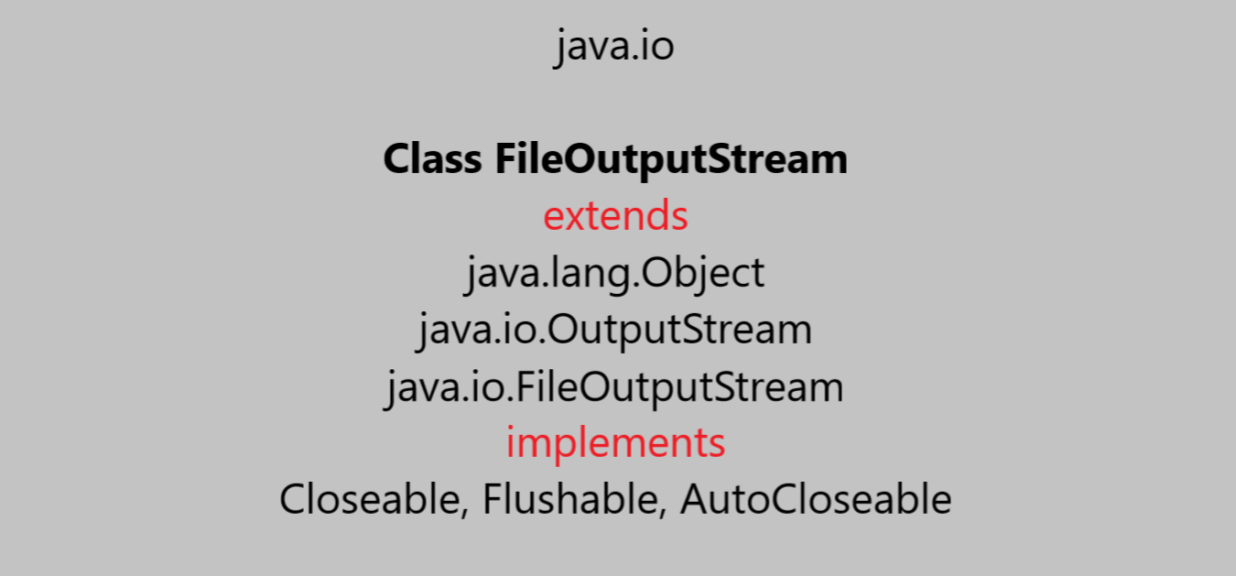
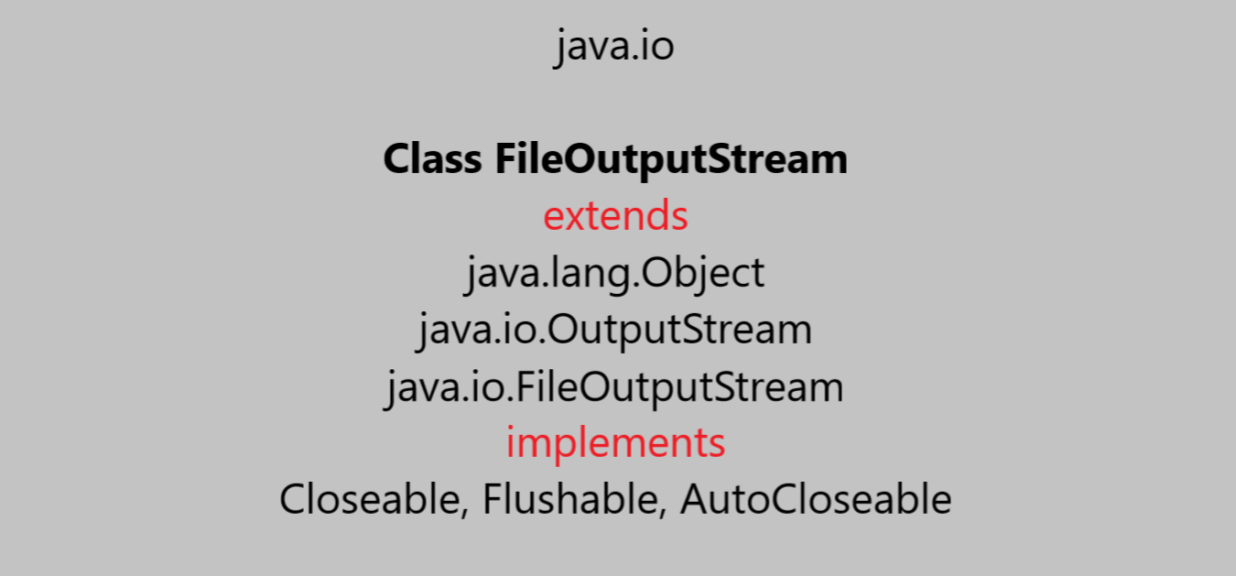
Example : Write to file line by line using FileOutputStream class
// Java Program for Write to file line by line using FileOutputStream class import java.io.File; import java.io.FileOutputStream; public class ExampleJavaFileWrite { public static void main(String[] args) { //Declaring reference of File class File file = null; //Declaring reference of FileOutputStream class FileOutputStream fileOutStream = null; String data = "TechBlogStation"; try { file = new File("D:/TBS/file.txt"); //Creating Object of FileOutputStream class fileOutStream = new FileOutputStream(file); //In case file does not exists, Create the file if (!file.exists()) { file.createNewFile(); } //fetching the bytes from data String byte[] b = data.getBytes(); //Writing to the file fileOutStream.write(b); //Flushing the stream fileOutStream.flush(); //Closing the stream fileOutStream.close(); System.out.println("File writing done."); } //Handing Exception catch (Exception e) { e.printStackTrace(); } finally { try { if (fileOutStream != null) { fileOutStream.close(); } } catch (Exception e) { e.printStackTrace(); } } } }
Java write to file line by line using FileWriter class
FileWriter class creates a writer which can be used to write to a file line by line. FileWriter class is part of the java.io package and it is a subclass of Writer class.


FileWriter creation is not dependent on whether file exists or not . In case file does not exist, FileWriter class will create the file before opening it for output at the time of object creation. In the case , where you are attempting to open a read-only file , an IO Exception will be thrown.
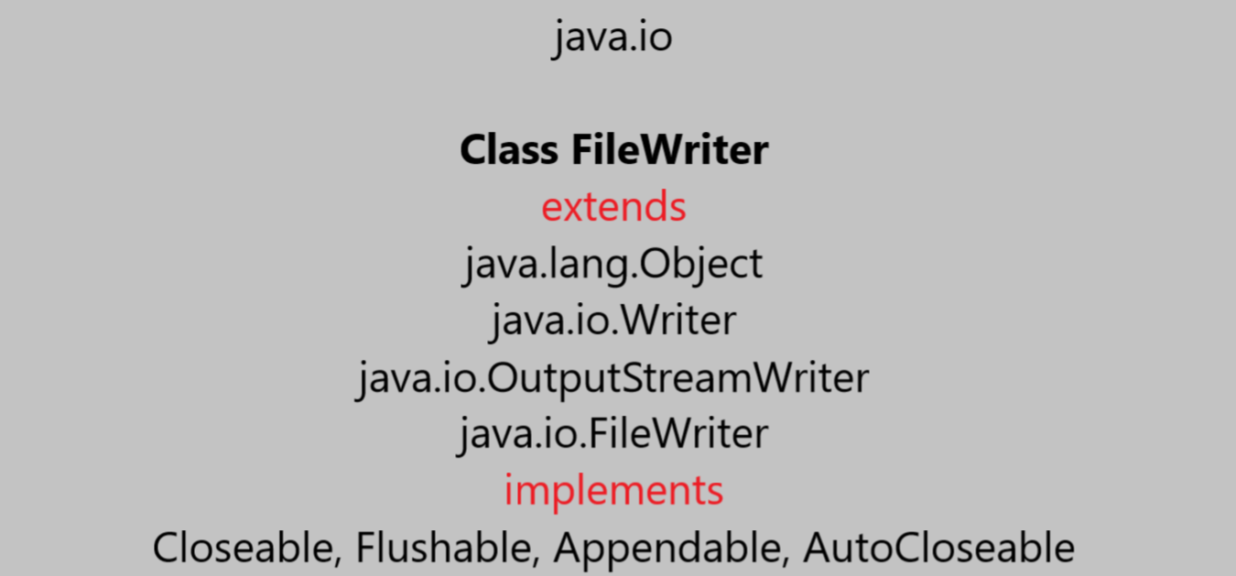
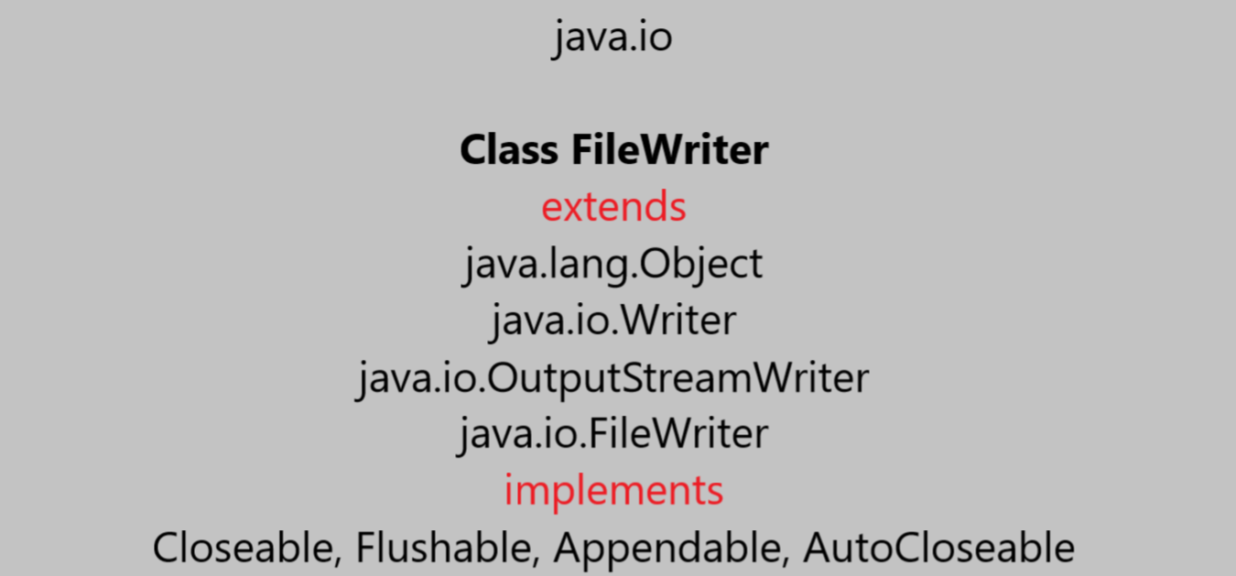
Example : Write to file line by line using FileWriter class
// Java Program for Write to file line by line using FileWriter class import java.io.File; import java.io.FileWriter; public class ExampleJavaFileWrite { public static void main(String[] args) { //Declaring reference of File class File file = null; //Declaring reference of FileWriter class FileWriter filewriter = null; String data = "TechBlogStation"; try { file = new File("D:/TBS/file.txt"); //Creating Object of FileWriter class filewriter = new FileWriter(file); //Writing to the file filewriter.write(data); //Closing the stream filewriter.close(); System.out.println("File writing done."); } //Handing Exception catch (Exception e) { e.printStackTrace(); } finally { try { if (filewriter != null) { filewriter.close(); } } catch (Exception e) { e.printStackTrace(); } } } }
Java write to file line by line using PrintWriter class
PrintWriter is a character oriented version of PrintStream class. Javaβs PrintWriter object supports both the print() and println() method for all types even including object. If the calling argument is not a simple type, the printwriter will call the objectβs toString() method and then print out the output.
PrintWriter class is part of the java.io package and it is a subclass of Writer class.
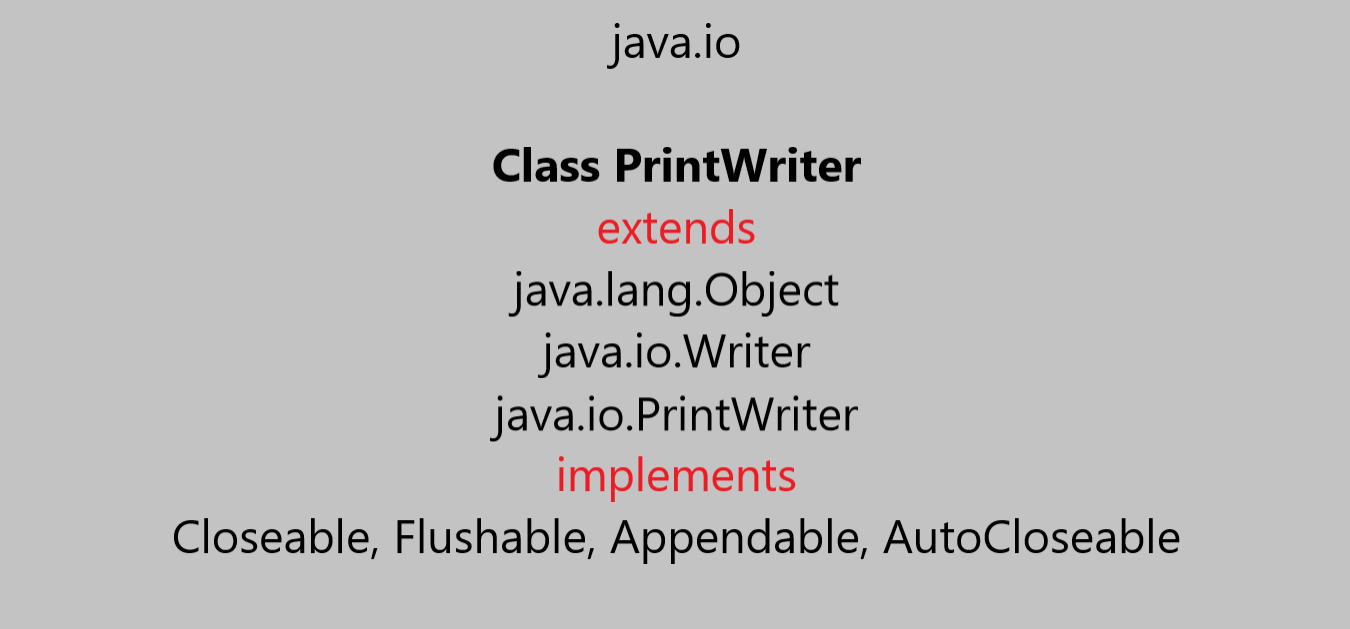
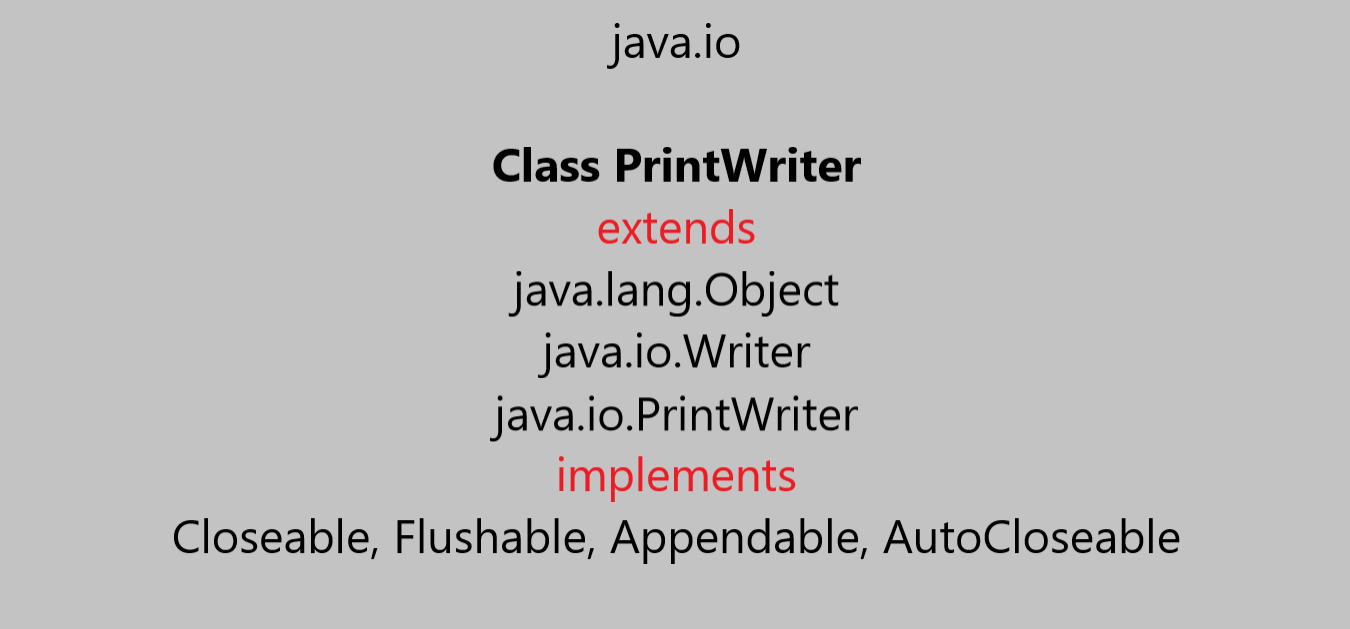
Example : Write to file line by line using PrintWriter class
// Java Program for Write to file line by line using PrintWriter class import java.io.File; import java.io.PrintWriter; import java.io.FileWriter; public class ExampleJavaFileWrite { public static void main(String[] args) { //Declaring reference of File class File file = null; PrintWriter pw = null; try { //Creating object of PrintWriter class pw = new PrintWriter(new FileWriter("file.txt")); String data = "TechBlogStation"; //Writing to the file pw.write(data); //Closing the stream pw.close(); System.out.println("File writing done."); } //Handing Exception catch (Exception e) { e.printStackTrace(); } finally { try { if (pw != null) { pw.close(); } } catch (Exception e) { e.printStackTrace(); } } } }
OutputStreamWriter class
An OutputStreamWriter acts as a bridge from character streams to byte streams: Characters written to it will be encoded into bytes using a charset
OutputStreamWriter class is part of the java.io package and it is a subclass of Writer class.

OutputStreamWriter class Example:
// Java Program for Write to file line by line using OutputStreamWriter class import java.io.File; import java.io.FileOutputStream; import java.io.OutputStreamWriter; public class ExampleJavaFileWrite { public static void main(String[] args) { //Declaring reference of File class File file = null; //Declaring reference of FileOutputStream class FileOutputStream fos = null; //Declaring reference of OutputStreamWriter class OutputStreamWriter osw = null; try { file = new File("result.txt"); //Creating object of FileOutputStream class fos = new FileOutputStream(file); //Creating object of OutputStreamWriter class osw = new OutputStreamWriter(fos); String data = "TechBlogStation"; //Writing to the file osw.write(data); //Closing the stream osw.close(); System.out.println("File writing done."); } //Handing Exception catch (Exception e) { e.printStackTrace(); } finally { try { if (osw != null) { osw.close(); } } catch (Exception e) { e.printStackTrace(); } } } }
Java write to file line by line using BufferedWriter
It will write text to a character-output stream, buffering characters to provide for the effective writing of single characters and strings.
A BufferedWriter is a Writer that adds a flush method which can be used to ensure that the data buffers are physically written to the actual output stream. BufferedWriter can increase the performance by reducing the number of times data is written to output stream.
BufferedWriter class is part of the java.io package and it is a subclass of Writer class.

Example : Write to file line by line using BufferedWriter
// Java Program for Write to file line by line using BufferedWriter class import java.io.File; import java.io.FileWriter; import java.io.BufferedWriter; public class ExampleJavaFileWrite { public static void main(String[] args) { //Declaring reference of File class File file = null; //Declaring reference of BufferedWriter class BufferedWriter bw = null; //Declaring reference of FileWriter class FileWriter fw = null; try { file = new File("result.txt"); //Creating object of FileWriter class fw = new FileWriter(file); //Creating object of BufferedWriter class bw = new BufferedWriter(fw); String data = "TechBlogStation"; //Writing to the file bw.write(data); //Closing the stream bw.close(); System.out.println("File writing done..."); } //Handing Exception catch (Exception e) { e.printStackTrace(); } finally { try { if (bw != null) { bw.close(); } } catch (Exception e) { e.printStackTrace(); } } } }
You can download the code for above examples from my github repository.
Conclusion
We have learnt about various ways of writing file line by line in Java with the Examples. Any of the way can be used as per requirement to write to file. Now it will be easy to write a program for you using any of these ways.