In this article, we will learn the topic Java Array Length. Java Arrays are very important in terms of Programming language.
We will understand its importance and different topics related to Java Array Length.
First , Let’s understand What are Java Arrays :
Introduction to Java Arrays
Array is a collection of similar type of elements. These elements have a contiguous memory location.
In Java, an array is an Object as everything in Java in an Object. Similarly, It stores elements of similar data type.
For example : A array of String will only contain String.
In Java array, all the elements are stored in contiguous memory location. Java Array can be seen as a data type containing similar type of elements.
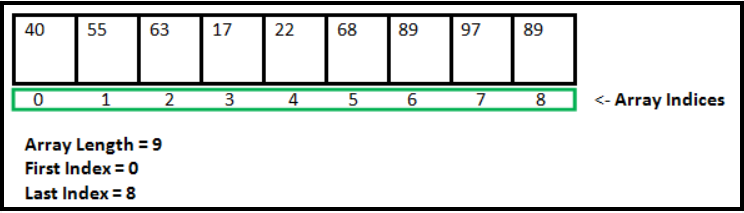
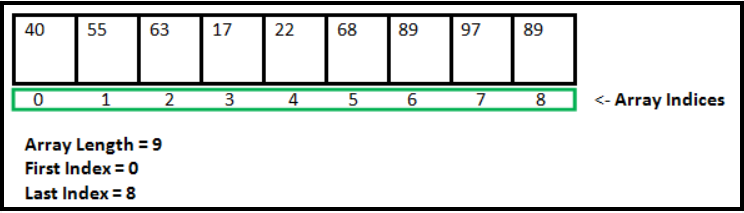
Java array allows only to store the limited number of elements. For example, if a Java Array is declared with the limit of 10, then it can only store 10 elements.
Java Arrays are index based data structure and the index starts from 0. First element will be stored in 0th position / index, seconds is in 1st position and so on.
Java Array Length
Java Array as we know is an Object. Java Array Object does not have a method which will return the length of the Java Array.
Oracle says, In Java an Array has a field “public final length” which denoted the number of element of the array.


Array Length Attribute
Java Array attribute which denoted length of array can range from zero to any positive number.
To get the Length or number of elements of Java Array , we need to use the array length attribute.
Here is a simple example to create a Java Array and to get the Java Array Length :
public class JavaArrayLength{ public static void main(String[] args) { //Creating array String[] names = { "Rachel", "Ross", "Phoebe" , "Monica"}; //Getting the Java Array Length int length = names.length; System.out.println("The length of the names array is: " + length); } }
Since the above names array has only four elements.
Below will be the output of Program :
The length of the names array is: 4
We can see in the above example, we have used Java Array Length attribute to get the array length / size as there is no method exists in Java array to check the same.
int length= names.length;
Above example was very simple but most of the time in the larger applications, we do not know how the object of array was created and our code becomes little complicated.
So let’s look at below example where we are writing separate function to receive an array as input and prints the length of the array as output.
Below is the Example :
public class JavaArrayLength { private static void printTheLength(String[] arr) { if (arr == null) { System.out.println("The Array is null so we can not determine the Java Array Length."); } else { int length = arr.length; System.out.println("The length of the input array is: " + length); } } public static void main(String[] args) { String[] array1= { "1", "2", "3" }; String[] array2= { "Tech", "Blog" }; String[] array3= { "Station" }; String[] array4= { "Testing" }; printTheLength (null); printTheLength (array1); printTheLength (array2); printTheLength (array3); printTheLength (array4); } }
In above printTheLength() , firstly we are checking if the provided input is null or not.
This is done to avoid NullPointerException in case of null object is passed.
Below is the output of above program:
The Array is null so we can not determine the Java Array Length. The length of the input array is: 3 The length of the input array is: 2 The length of the input array is: 1 The length of the input array is: 1
Searching for a Value using Java Array Length
The Java array length has various properties which are very useful and easy to use.
Suppose if you want to loop through the complete array and want to display all its elements or want to perform any operation on all of its element or simply wants to search any element in the Java Array.
Then we can use the Java Array Length property. We can use this property to loop through each element present in the java array.
See Below example where we are looping each element of Java Array using Java Array Length property to search for an element :
public class ArrayLength {
//Function to search the element in the entire Java Array
private static boolean check(String[] arr, String val) {
if (arr != null) {
int length = arr.length;
for (int i = 0; i <= length - 1; i++) {
String value = arr[i];
if (value.equals(val)) {
return true;
}
}
}
return false;
}
public static void main(String[] args) {
String[] arr = { "Tech", "Blog", "Station" };
System.out.println(check(arr, "Blog"));
System.out.println(check(arr, "Tech"));
}
}
In the above example, first we are checking if array is not null then we are looking for the element to search in the entire array.
You can see, we have used the Java Array Length attribute to loop through an entire array :
for (int i = 0; i <= length - 1; i++)
**Where length is the array length
The Output of The Program will be :
true true
Searching for Lowest Value in Array using Java Array Length
We can use the Java Array Length to find the lowest element exists in the Java array object.
See the below example, where we are using Java Array Length attribute to find the lowest element of Java Array :
public class ArrayLength {
//Method to find the minimum value
private static int findMin(int[] arr) {
int minVal = arr[0];
int length = arr.length;
for (int i = 1; i <= length - 1; i++) {
int value = arr[i];
if (value < minVal) {
minVal = value;
}
}
return minVal;
}
public static void main(String[] args) {
int[] arr = { 5, 4, 2, 3, 6 };
System.out.println("The minimum value in the array is: " + findMin(arr));
}
}
We have used Java Array Length in above example to loop through the array to find the minimum value present in the Java Array Object.
The Output of the above program will be :
The minimum value in the array is: 2
Searching for Highest Value in Array using Java Array Length
We can use the Java Array Length to find the highest element exists in the Java array object.
See the below example, where we are using Java Array Length attribute to find the highest element of Java Array :
public class ArrayLength {
//Method to find the maximum value
private static int findMax(int[] arr) {
int maxVal = arr[0];
int length = arr.length;
for (int i = 1; i <= length - 1; i++) {
int value = arr[i];
if (value > maxVal) {
maxVal = value;
}
}
return maxVal;
}
public static void main(String[] args) {
int[] arr = { 5, 4, 2, 3, 6 };
System.out.println("The maximum value in the array is: " + findMax(arr));
}
}
We have used Java Array Length in above example to loop through the array to find the maximum value present in the Java Array Object.
The Output of the above program will be :
The maximum value in the array is: 6
Conclusion
In this article, we have learnt about the Java Array , Its different examples and learnt How to use Java Array Length for different purpose with examples.