Introduction
In this article, we will learn this keyword in Java and its each usage with Examples.
this is a keyword in Java. It is a reference variable and it refers to the current object.


It has multiple usage in Java, which we will learn in next section.
Usage of this keyword in Java
Java this keyword can be used for below mentioned six purposes :
- this keyword in Java is used to refer the current class object.
- this keyword is used to implicitly invoke the method of current class.
- this is used in Java to invoke current class constructor.
- this in Java is used as argument as we can pass it in method call.
- this in Java is used as argument for constructor.
- this in Java is used to return the current class instance from any method.


Now, Let’s understand each usage of this keyword in detail :
this: to refer current class object
this keyword in Java is used to refer the current class instance variable / object.
It is used to resolve ambiguity issues between the instance variables and parameters.
As Object Oriented Programming is very similar to real life that’s why it is always recommended to name the variables and parameters appropriately as real time.
Thus , the instance variable name and parameter might be same many times which leads to ambiguity.
Hence, we use ‘this’ keyword to solve this problem.
Let’s understand the problem without using this keyword :
class Employee{
int id;
String name;
float salary;
Employee(int id,String name,float salary){
id=id;
name=name;
salary=salary;
}
void view(){
System.out.println(id+" "+name+" "+salary);
}
}
public class MainClass{
public static void main(String args[]){
Employee obj1 = new Employee(15,"Chandler",30000f);
Employee obj2 = new Employee(18,"Joey",45000f);
obj1.view();
obj2.view();
}}
Output :
0 null 0.0 0 null 0.0
As we can see in the above example, the name of instance variables and parameters is same. So compiler is confused that’s why we use this keyword in Java to solve this problem of ambiguity.
Let’s see the solution of this problem :
Example : Program of this keyword to refer the current class instance variable
class Employee{
int id;
String name;
float salary;
Employee(int id,String name,float salary){
this.id=id;
this.name=name;
this.salary=salary;
}
void view(){
System.out.println(id+" "+name+" "+salary);
}
}
public class MainClass{
public static void main(String args[]){
Employee obj1 = new Employee(15,"Chandler",30000f);
Employee obj2 = new Employee(18,"Joey",45000f);
obj1.view();
obj2.view();
}}
Output :
15 Chandler 30000.0 18 Joey 45000.0
this: to invoke current class method
this keyword in Java is also used for invoking the current class method.
The Java compiler always by default add the this keyword everytime we invoke a method.
See the below diagram :
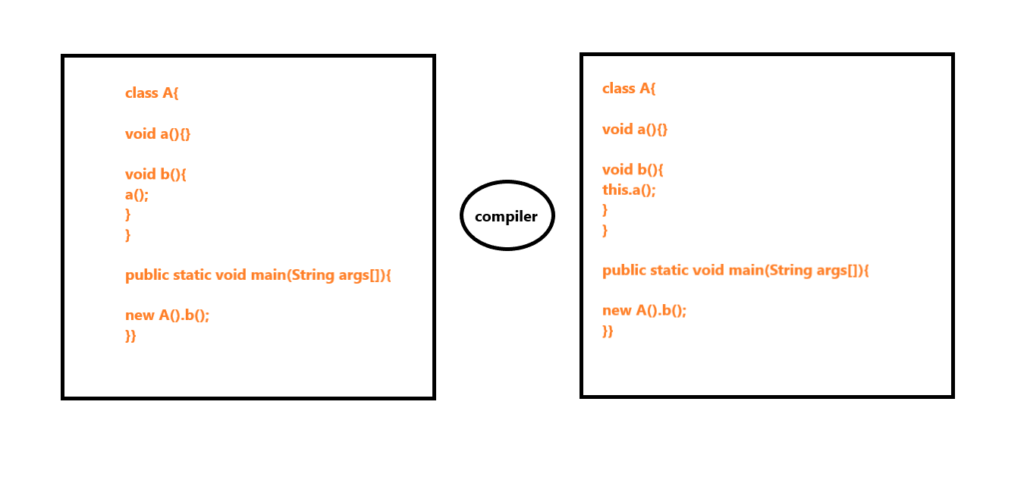
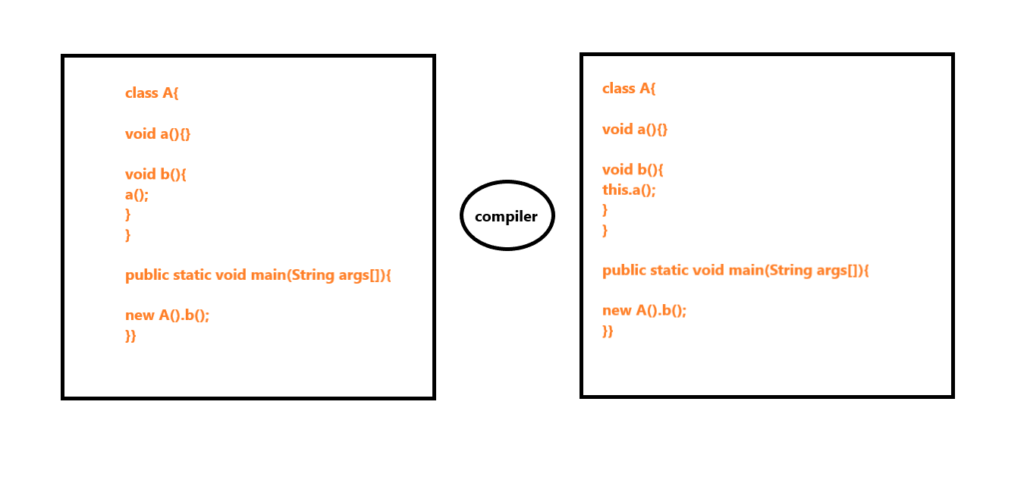
Example : Program of this keyword in java to invoke the current class method
class Test{
void one(){
System.out.println("Method one");
}
void two(){
System.out.println("Method two");
this.one();
}
}
public class Sample{
public static void main(String args[]){
Test obj = new Test();
obj.two();
}}
Output :
Method two Method one
this: to invoke current class constructor
We can use this() to call the constructor in Java. This will invoke the constructor.
This way, we can reuse the constructor in Java. This concept is known as constructor chaining.
Constructor chaining is the concept where the constructor of a class is being called from another constructor of same class. It build a chain between the constructors.
The most important thing is to remember that – “Call to this() must be the first statement in constructor.“
Example : Program of this keyword to invoke the current class constructor (constructor chaining example)
class Employee{
int id;
String name;
float salary;
String department;
Employee(int id,String name,float salary){
this.id=id;
this.name=name;
this.salary=salary;
}
Employee(int id,String name,float salary,String department){
this(id,name,salary); //Invoking the constructor
this.department=department;
}
void view(){
System.out.println(id+" "+name+" "+salary+" "+department);
}
}
public class MainClass{
public static void main(String args[]){
Employee obj1 = new Employee(15,"Chandler",30000f,"Statistical");
Employee obj2 = new Employee(18,"Joey",45000f);
obj1.view();
obj2.view();
}}
Output :
15 Chandler 30000.0 Statistical 18 Joey 45000.0 null
Q. Will the below Program Execute ?
class Employee{
int id;
String name;
float salary;
String department;
Employee(int id,String name,float salary){
this.id=id;
this.name=name;
this.salary=salary;
}
Employee(int id,String name,float salary,String department){
this.department=department;
this(id,name,salary); //Compile Time error
}
void view(){
System.out.println(id+" "+name+" "+salary+" "+department);
}
}
public class MainClass{
public static void main(String args[]){
Employee obj1 = new Employee(15,"Chandler",30000f,"Statistical");
Employee obj2 = new Employee(18,"Joey",45000f);
obj1.view();
obj2.view();
}}
Above program will not get compiled as this() must be the first statement in the constructor.
Output :
MainClass.java:20: error: call to this must be first statement in constructor this(id,name,salary); ^ 1 error
this: to pass as an argument in method
We can pass this keyword in Java as the argument of a method.
this keyword is used in argument of method mainly for event handling. In the event handling , we may require to provide reference of a class to another one. There, we can use this keyword as an argument in the method.
Let’s take a look at below Example :
Example : Program of this keyword in java passed as argument in method
public class A{
void one(A object){
System.out.println("Method one is invoked");
}
void two(){
one(this);
}
public static void main(String args[]){
A object1 = new A();
object1.two();
}
}
Output :
Method one is invoked
this: to pass as an argument in constructor
this keyword can be passed in java constructor as well.
In case , where we are required to use one object of a class in multiple classes then we use this in Java as the constructor argument.
Let’s see the below example :
Example : Program of this keyword in java passed as argument in constructor
class A{
B object;
A(B object){
this.object=object;
}
void view(){
System.out.println(""+object.name);
}
}
public class B{
String name="Rachel";
B(){
A obj = new A(this);
obj.view();
}
public static void main(String args[]){
B b=new B();
}
}
Output :
Rachel
this: to return current class object
We can use ‘this’ keyword as return statement of a method in Java.
In these scenarios, the return type of the method must be the class type and nothing else.
Let’s see the below syntax:
return_typeΒ method_name(){Β Β returnΒ this;Β Β }Β Β //return_type must be class name
Example : Program to use this keyword in java to return class object
class Test{
Test getTest(){
return this;
}
void data(){
System.out.println("I love F.R.I.E.N.D.S");
}
}
public class Sample{
public static void main(String args[]){
new Test().getTest().data();
}
}
Output :
I love F.R.I.E.N.D.S
Proof of working of this keyword in Java
Now, we will prove that this in java refers to the current class object.
In the below example, we are trying to print the reference variable and also the this. If Output of both are same, that means this refers to the current class instance variable.
public class Test{
void printing(){
System.out.println(this);
}
public static void main(String args[]){
Test obj = new Test();
System.out.println(obj);
obj.printing();
}
}
Output :
Test@6d06d69c Test@6d06d69c
Conclusion
Let’s summarize the whole concept explained above :
- Java ‘this’ is used as a reference variable which refers to the current object.
- ‘this’ is used to refer current class instance variable in java.
- ‘this’ can be used for invoking the constructor.
- ‘this’ can be used as a method argument.
- ‘this’ can be used as a constructor argument.
- ‘this’ can be used for return the current class instance.
- ‘this’ avoids naming conflicts.
Thanks for Reading!