Introduction
Method overloading in Java is a concept where a class can have methods with same name but different parameters.
This concept improves the readability.
As we know, Object oriented Programming is very similar to real life so the names of methods , variables should be real time.
Hence, Suppose a method is performing a sum operation then we should name the method sum.
Now, if your class has two methods for addition; one where two digits are being added and second where three digits are being added.
So to improve readability, we should name both the methods as sum. This is exactly called as method overloading in java.
Advantages of Method Overloading
There are basically two main advantages of Method Overloading, which are mentioned below :
- It improves readability.
- It is used to achieve compile time polymorphism.
Ways to achieve Method Overloading in Java
In Java, we can achieve method overloading by using any of below three ways :
1) different number of parameters.
Example : sum(int, int) sum(int, int, int)
2) different data type of parameters.
Example : sum(int, float) sum(int, int)
3) different sequence of data type of parameters.
Example : sum(int, float) sum(float, int)
What is not Method Overloading in Java?
We cannot achieve method overloading in Java by changing the return type of method.
Suppose, if a class has two methods with same name, same parameters but different return type, as defined below :
int sum(int, int)
float sum(int, int)
It is Invalid.
The reason is simple. Method Overloading is a compile time polymorphism and a static binding technique in Java.
It is decided at compile time that which method is invoked and return value of any method is determined at run time only.
Thus, Compiler will get confused in the above scenario. Thatβs why it is not way of achieving method overloading in Java.
Compiler throws exception in such cases.
Method Overloading Examples in Java
Now, Letβs look at different examples showing different ways to achieve method overloading.
Example 1: Changing number of Parameters
public class MethodOverLoadEx{
//Method 1
int sum(int x,int y){
return x+y;
}
//Method 2
int sum(int x,int y,int z){
return x+y+z;
}
public static void main(String[] args){
MethodOverLoadEx obj = new MethodOverLoadEx();
System.out.println(obj.sum(20,30));
System.out.println(obj.sum(20,30,40));
}}
Output :
50 90
In the above example, we have two methods sum(int,int) and sum(int,int,int).
When we are invoking sum method with two int parameters then the first one is being called and when with three int parameters then the second one is being invoked.
Example 2: Changing data type of Parameters
public class MethodOverLoadEx{
//Method 1
double sum(int x,double y){
return x+y;
}
//Method 2
int sum(int x,int y){
return x+y;
}
public static void main(String[] args){
MethodOverLoadEx obj = new MethodOverLoadEx();
System.out.println(obj.sum(20,30));
System.out.println(obj.sum(20,30.2));
}}
Output:
50 50.2
In the above example, we have two methods sum(int,int) and sum(int,double).
When we are invoking sum method with int parameters then the first one is being called and when with one int and one double parameter then the second one is being invoked.
Example 3: Changing sequence of Parameters
public class MethodOverLoadEx{
//Method 1
double sum(int x,double y){
return x+y;
}
//Method 2
double sum(double x,int y){
return x+y;
}
public static void main(String[] args){
MethodOverLoadEx obj = new MethodOverLoadEx();
System.out.println(obj.sum(30,30.2));
System.out.println(obj.sum(50.2,20));
}}
Output :
60.2 70.2
Method Overloading and Type Promotion
Type promotion in Java is a concept where one type is promoted to another bigger type in case the matching data type is found.
Below Diagram shows, which type can be promoted to which type :

As per above figure,
- byte can be promoted to int, short, long, float and double.
- short can be promoted to int, long, float and double.
- int can be promoted to long, float and double.
- char can be promoted to int, long, float and double.
- so onβ¦
Example 1: Method Overloading with Type Promotion : Match not found
public class MethodOverLoadEx{
//Method 1
long sum(int x,long y){
return x+y;
}
//Method 2
int sum(int x,int y,int z){
return x+y;
}
public static void main(String[] args){
MethodOverLoadEx obj = new MethodOverLoadEx();
System.out.println(obj.sum(30,10)); //Now, here second parameter is int but it will be type
//promoted to long
System.out.println(obj.sum(50,20,30));
}}
Output:
40 70
We can see in above example, we passed int in sum(int,long) but the int is automatically type promoted to long as there exists no matching method for sum(int, int).
Now, Letβs see what happens if the matching method is present in the class:
Example 2: Method Overloading with Type Promotion : Match found
public class MethodOverLoadEx{
//Method 1
int sum(int x,int y){
System.out.println("int, int method invoked...");
return x+y;
}
//Method 2
long sum(long x,long y){
System.out.println("long, long method invoked...");
return x+y;
}
public static void main(String[] args){
MethodOverLoadEx obj = new MethodOverLoadEx();
System.out.println(obj.sum(30,10));
}}
Output:
int, int method invoked... 40
Now, since the matching method is found type promotion is not performed.
Example 3: Method Overloading with Type Promotion : Ambiguity
public class MethodOverLoadEx{
//Method 1
long sum(int x,long y){
System.out.println("int, long method invoked...");
return x+y;
}
//Method 2
long sum(long x,int y){
System.out.println("long, int method invoked...");
return x+y;
}
public static void main(String[] args){
MethodOverLoadEx obj = new MethodOverLoadEx();
System.out.println(obj.sum(30,10));
}}
Output:
MethodOverLoadEx.java:22: error: reference to sum is ambiguous System.out.println(obj.sum(30,10)); ^ both method sum(int,long) in MethodOverLoadEx and method sum(long,int) in MethodOverLoadEx match 1 error
Since both the methods are not matching and both are applicable for type promotion so compiler will throw error as this is case of ambiguity.
Method Overloading vs Method Overriding
They both are used to achieve Polymorphism in Java.
Method Overloading achieves Compile time polymorphism in Java and Method overriding achieves Runtime polymorphism in Java.
See the below diagram :
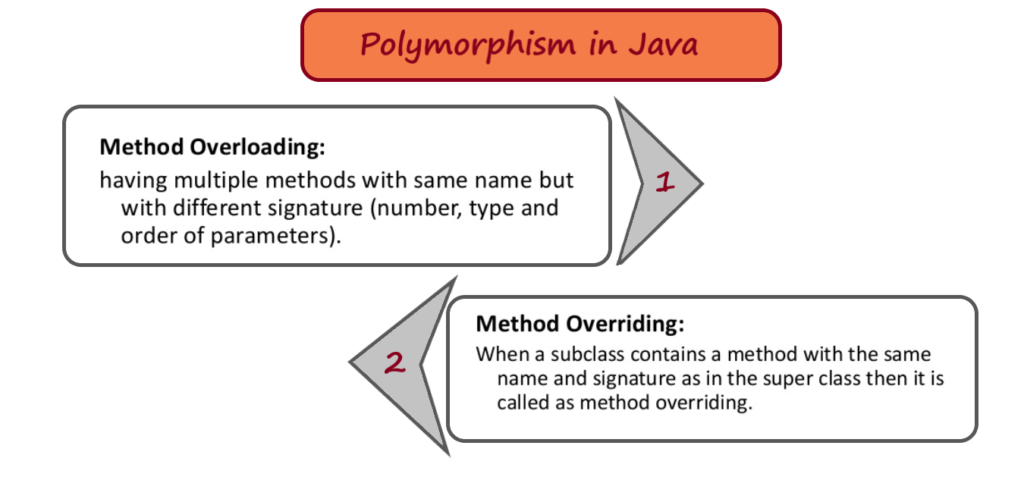
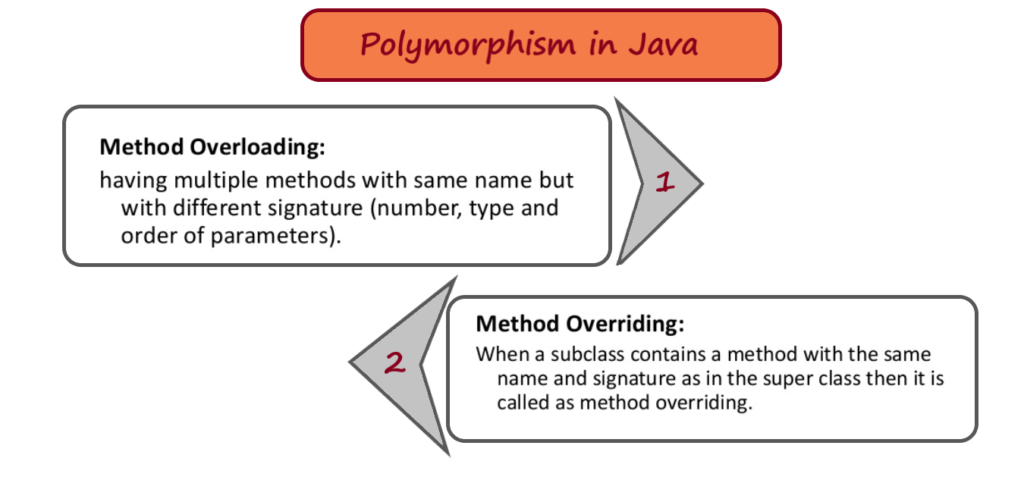
Frequently Asked Questions
Yes, we can overload main method in Java.
JVM will consider the main method, which will have String[] args as parameter, as the entry point of the class. Other method will behave like normal methods.
See the below code snippet to understand the result :public class Test{
public static void main(String[] args){
System.out.println("main - String[] args");
}
public static void main(String args){
System.out.println("main - String args");
}
public static void main(int args){
System.out.println("main - int args");
}
public static void main(){
System.out.println("main - no parameters");
}
}
Output:
main - String[] args
To improve Readability of the code and to achieve compile time polymorphism in Java.
It will lead to ambiguity. See the above section : here.
Further Readings :
Conclusion
Method Overloading in Java can be achieved by three ways. It has multiple advantages such as readability improvement etc.
It is a way to achieve compile time polymorphism in Java. It is one of the most important question of Java interview.
Thanks for Reading!