Introduction
Encapsulation in Java is a Object-Oriented-Programming concept. It is a technique of wrapping data and code together into a single unit.
Simple Example of Encapsulation in real life is – A Capsule, several medicines are wrapped into one outer layer.
In Java, class is the example of encapsulation.
Let’s learn in detail :
What is Encapsulation?
Encapsulation is wrapping code and data together into one single unit.
Encapsulation in java is performed using class. A class wraps code (method) and data(variables) together.
This concept ensures that data members of a class are always hidden from other classes. We can only access those data members using the methods.
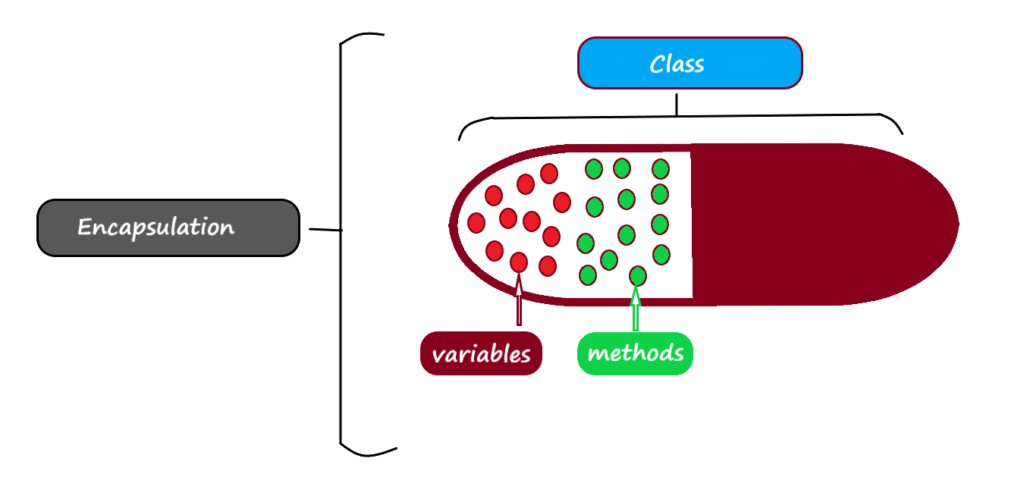
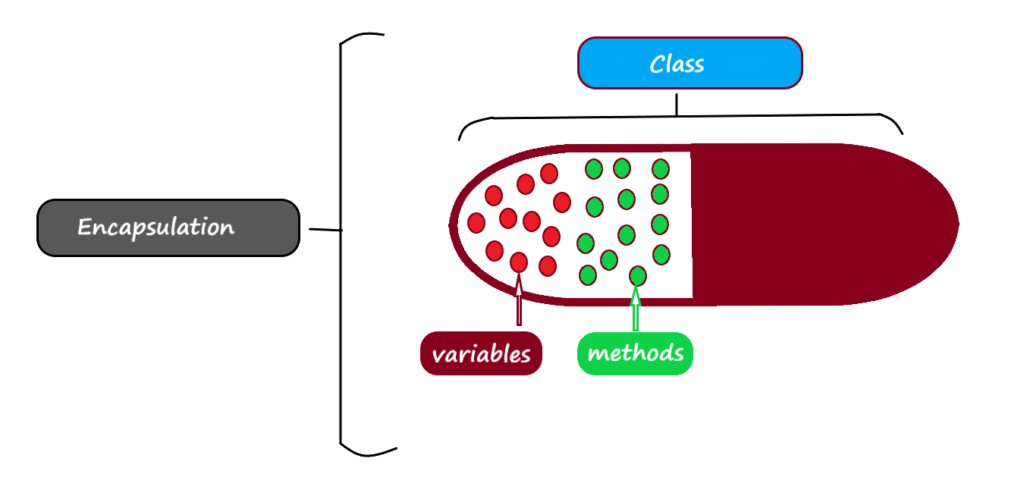
A class is the example of encapsulation concept. Java bean / POJO class is considered to be fully encapsulated class in Java. All the data members of these classes are private.
Encapsulation is also known as data-hiding as it prevents data to be accessed by the code outside the class.
Advantages of Encapsulation in Java
Encapsulation in java has many advantages, which are mentioned below :
- We can make read-only and write-only classes using encapsulation.
- We can achieve data hiding.
- The encapsulated classes are better for unit testing.
- It is easy and fast.
- We have control over data.
Example : Simple program of Encapsulation in Java
Below program has two classes – Employee
and Test
. This example illustrates simple encapsulation :
Employee.java
package com.tbs;
//It is a fully encapsulated class
public class Employee{
//data members are private
private String name;
private int emp_id;
//getter methods
public String getName(){
return name;
}
public int getEmp_id(){
return emp_id;
}
//setter methods
public void setEmp_id(int emp_id){
this.emp_id=emp_id;
}
public void setName(String name){
this.name=name;
}
}
Test.java
package com.tbs;
public class Test{
public static void main(String[] args){
Employee employee = new Employee();
//setting the values
employee.setName("Rahul");
employee.setEmp_id(100);
//getting the values
System.out.println("Name "+employee.getName());
System.out.println("Id "+employee.getEmp_id());
}
}
Output:
Name Rahul Id 100
Example : How to create Read only class in Java
Below are the steps to create read only classes in java using encapsulation :
- only private data members.
- no setter methods.
Below is the code to create read-only classes in Java. Other classes can not make any changes in the fields of these type of classes.
public class Employee{
private String name="Rohan";
public String getName(){
return name;
}
//No setter methods
}
Above class has private data member and does not have any setter method thus it is read-only class.
Example : How to create Write only class in Java using Encapsulation
We can create write only classes using encapsulation by following below steps in a class:
- only private data members.
- no getter methods.
Below is the code to create write-only classes in Java. classes can not read the value of this class’s fields.
public class Employee{
private String name;
public String setName(String name){
this.name = name;
}
//No getter methods
}
Above class has private data member and does not have any getter method thus it is write-only class.
Example : Another program of Encapsulation in Java
Employee.java
public class Employee {
private long emp_id;
private String emp_name;
private float salary;
//getter methods and setter methods
public long getEmp_id() {
return emp_id;
}
public void setEmp_id(long emp_id) {
this.emp_id = emp_id;
}
public String getEmp_name() {
return emp_name;
}
public void setEmp_name(String emp_name) {
this.emp_name = emp_name;
}
public float getSalary() {
return salary;
}
public void setSalary(float salary) {
this.salary = salary;
}
}
TestApp.java
public class TestApp {
public static void main(String[] args) {
Employee employee=new Employee();
employee.setEmp_id(1234);
employee.setEmp_name("James Bond");
employee.setSalary(35000f);
System.out.println(employee.getEmp_id()+" "+employee.getEmp_name()+" "+employee.getSalary());
}
}
Output:
1234 James Bond 35000.0
Encapsulation vs Abstraction
Encapsulation and Abstraction are two different topics but many get confused between both of them .
Let’s look at the difference :
- Abstraction deals with “What”.
- Encapsulation deals with “How”.
Abstraction hides the complex functionalities and encapsulation binds the functionalities.
Further Readings:
Conclusion
Encapsulation in java is a oops concept and it helps in data binding and hiding both.
It adds many advantages to Java such as facility to create read only and write only classes, data hiding etc.
It is a basic oops interview question for freshers and experienced both.
Thanks for Reading!